UnitTest for StreamWriter
[Test]
public void Method_Writing_To_Output_Stream()
{
// arrange
using (var stream = new MemoryStream())
using (var writer = new StreamWriter(stream))
{
// act
testObject.SomeMethod(writer);
// assert
string actual = Encoding.UTF8.GetString(stream.ToArray());
Assert.AreEqual("some expected output", actual);
}
}
Resetting StreamReader when using MemoryStream
I wanted to Unit test reading a file multiple times, to make sure everything was being cleared from previous reads. I was using the MemoryStream technique highlighted in the above note, but for reading rather than writing. My initial implementation simply created a new StreamReader on the same MemoryStream, but that didn’t work. The reason is that the MemoryStream is at the end and needs to be re-postioned to the start.
CVConfiguration cvConfig = new CVConfiguration();
// Create Config Lines.
StringBuilder buf = new StringBuilder();
buf.Append("Test Wave Frequency = 105.5 \n");
buf.Append("END of config \n");
MemoryStream config =
new MemoryStream(Encoding.ASCII.GetBytes(buf.ToString()));
StreamReader reader = new StreamReader(config);
cvConfig.LoadConfiguration(reader);
Assert.AreEqual(1, cvConfig.Frequency.Count);
Assert.AreEqual(105.5, cvConfig.Frequency[0]);
config.Position = 0;
reader.DiscardBufferedData();
cvConfig.LoadConfiguration(reader);
Assert.AreEqual(1, cvConfig.Frequency.Count);
Assert.AreEqual(105.5, cvConfig.Frequency[0]);
}
Argument Null Test Exception
_param = param ?? throw new ArgumentNullException(nameof(param));
Nunit – Check for Exceptions
It used to be that Exceptions thrown in Units tests were defined as a Test Attribute. However that was considered an ‘Anti-Pattern’ (WTF – It’s a Unit Test Function – what difference does it make!). Hoever as of Nunit 3, the mechanism for detecting Exceptions is to explicitly state that a call should trigger a specified type of exception.
Assert.That(() => function(params), Throws.TypeOf<Exception_Type>());
Remove Whitespace from Strings
string.Concat(line.Where(c => !char.IsWhiteSpace(c)))
Initialising a Dictionary with Annonymous functions
_commandExecutions = new Dictionary<ConfigCommandType, CommandExecution>()
{
{ConfigCommandType.StartVoltage, (data) => { StartVoltage = Double.Parse(data); } },
{ConfigCommandType.FinalVoltage, (data) => { FinalVoltage = Double.Parse(data); } },
{ConfigCommandType.Frequency, (data) => AddTestWaveFrequency(data) },
{ConfigCommandType.Comment, (data) => Comment = data },
{ConfigCommandType.End, (data) => EndComment = data }
};
Assembly Version Info
The Assembly version string is specified in the Project Properties, and can be viewed and edited either by examining the AssembltInfo.cs file under Properties, or by opening the selecting the Application tab in the Project Properties and clicking the Assembly Information… button
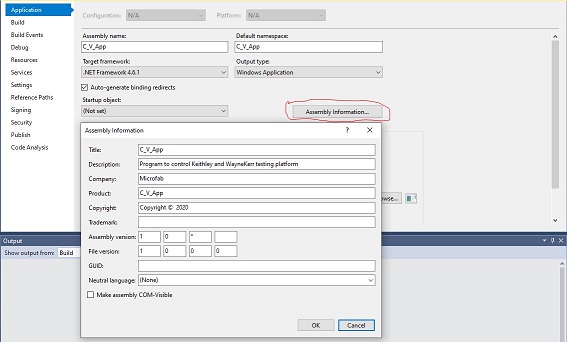
If you want to have the Version to update automaically on every build, then set the third digit to ‘*’. You will also need to edit the .csproj project file, and change set <Deterministic> property (located in the first <PropertyGroup> element) to false. It is set to true by default.
Access Version Info from C# App:
An App can (and often will) be comprised of several projects, and hence multiple assemblies. If you want the Version of the Initial Assembly then:
using System.Reflection;
string version = Assembly.GetEntryAssembly().GetName().Version.ToString()
To get the version of the Assembly that is being executed:
using System.Reflection;
string version = Assembly.GetExecutingAssembly().GetName().Version.ToString()